학습할 것 (필수)
선택문
반복문
JUnit 5 학습하세요.
- 인텔리J, 이클립스, VS Code에서 JUnit 5로 테스트 코드 작성하는 방법에 익숙해 질 것.
- 이미 JUnit 알고 계신분들은 다른 것 아무거나!
live-study 대시 보드를 만드는 코드를 작성하세요.
- 깃헙 이슈 1번부터 18번까지 댓글을 순회하며 댓글을 남긴 사용자를 체크 할 것.
- 참여율을 계산하세요. 총 18회에 중에 몇 %를 참여했는지 소숫점 두자리가지 보여줄 것.
- Github 자바 라이브러리를 사용하면 편리합니다.
- 깃헙 API를 익명으로 호출하는데 제한이 있기 때문에 본인의 깃헙 프로젝트에 이슈를 만들고 테스트를 하시면 더 자주 테스트할 수 있습니다.
LinkedList를 구현하세요.
- LinkedList에 대해 공부하세요.
- 정수를 저장하는 ListNode 클래스를 구현하세요.
- ListNode add(ListNode head, ListNode nodeToAdd, int position)를 구현하세요.
- ListNode remove(ListNode head, int positionToRemove)를 구현하세요.
- boolean contains(ListNode head, ListNode nodeTocheck)를 구현하세요.
Stack을 구현하세요.
- int 배열을 사용해서 정수를 저장하는 Stack을 구현하세요.
- void push(int data)를 구현하세요.
- int pop()을 구현하세요.
앞서 만든 ListNode를 사용해서 Stack을 구현하세요.
- ListNode head를 가지고 있는 ListNodeStack 클래스를 구현하세요.
- void push(int data)를 구현하세요.
- int pop()을 구현하세요.
Queue를 구현하세요.
- 배열을 사용해서 한번
- ListNode를 사용해서 한번.
선택문
-
골라 뽑는 것. 선탁(選擢).
[출처 : 옥스퍼드 사전]
뜻 그대로 여럿 가운데서 필요한것을 고르는 것을 선택이라고 한다.
자바에서 선택문이란 조건이 주어지고 그 조건에 해당하는 프로세스를 실행한다.
키워드는 if , else if, else , switch 가 있다.
if,else if, else
void ifTest(){
String myFavoriteColor = "purple";
if(myFavoriteColor.equals("red")){
System.out.println("RED IS NOT MY FAVORITE COLOR");
}else if(myFavoriteColor.equals("blue")){
System.out.println("BLUE IS NOT MY FAVORITE COLOR");
}else if(myFavoriteColor.equals("purple")){
System.out.println("PURPLE IS MY FAVORITE COLOR");
}else{
System.out.println("WHATEVER");
}
}
해당 테스트에서 첫번째 선택지인 if(myFavoriteColor.equals("red")로 들어왔다가 해당 조건이 참이면 중괄호로 묶여진 스코프를 실행하게 된다.
두번째 선택지인 else if는 전의 조건이 참이 아닐경우 다른조건을 제시하는 문장이다. 첫번째 조건인 "좋아하는 색은 빨강인가?" 가 거짓이기 때문에 else if구문을 한번 더 확인하게 된다. 두번째 구문인 "좋아하는 색은 파랑인가?" 의 경우도 거짓이기 때문에 해당 스코프는 실행되지 않는다.
if와 else if의 조건이 모두 거짓일 경우 else가 실행되게 된다.
switch
void switchTest(){
String myFavoriteAnimal = "cat";
switch (myFavoriteAnimal){
case "dog" -> System.out.println("NOPE");
case "cat" -> System.out.println("YES");
default -> System.out.println("I don't like animal");
}
}
해당 테스트에서는 switch조건을 통해 내가 제시한 조건과 일치하는 스코프를 실행시킨다.
case 별로 들어온 조건일 경우 다른 프로세스를 실행할 수있다.
+ 자바 13부터 스위치 람다가 가능하다고한다. 이번에하면서 처음알았네;
반복문
반복문이랑 어느 조건을 주고 해당조건이 거짓이 될때까지 반복되는 문장을 말한다.
void forTest(){
for (int i = 0; i < 10; i++) {
System.out.println(i+1+"번째 반복");
}
}
해당 반복문은 변수i가 10보다 작다 라는 조건이 걸려있고 해당 스코프가 실행될 때 마다 i를 후처리 증감연산을 하겠다는 의미이다.
그 밖에 반복문으로 while문이 있다.
for문 , while문에 는 사용방법이 다양하게 있으니 관심있으면 찾아보도록 하자.
예
ArrayList<Integer> intArray = new ArrayList<Integer>();
intArray.forEach(integer -> {
});
for (int integer: intArray) {
}
JUnit 5 학습하세요.
The 5th major version of the programmer-friendly testing framework for Java and the JVM
JUnit 5
The JUnit team uses GitHub for version control, project management, and CI.
junit.org
JUnit5 사이트에 나오는 설명문구이다.
말 그대로 Java와JVM의 프로그래머 진화적인 테스트프레임워크의 다섯번째 메이저 버전이다.
JUnit은 테스트 프레임워크 로 요즘 강조되고있는 TestCode를 작성하고 실행할 수 있는 환경을 말한다.
자세한 내용은 공식문서를 읽어보면 좋겠지만 , 영어라서 해석하는데 꽤나 걸리기 때문에 간략하게 정리하겠다.
특징
- 테스트를 작성하고 실행하는 데 사용되는 오픈 소스 프레임 워크
- annotation을 제공
- 테스트 기대 결과를 위한 assertion 제공
- test runner를 제공한다.
JUnit 5 = JUnit Platform + JUnit Jupiter + JUnit Vintage
JUnit Platform
JUnit 플랫폼은 JVM에서 테스트 프레임워크를 시작하기 위한 기반 역할을 한다. 또한 플랫폼에서 실행되는 테스트 프레임워크를 개발하기 위한 테스트 엔진 API를 정의합니다. 또한, 플랫폼은 명령행에서 플랫폼을 시작할 수 있는 콘솔 런처와 플랫폼에서 하나 이상의 테스트 엔진을 사용하여 사용자 지정 테스트 스위트를 실행할 수 있는 JUnit Platform Suite Engine을 제공한다. JUnit 플랫폼에 대한 1등급 지원은 인기 있는 IDE(인텔리J IDEA, 이클립스, 넷빈스, 비주얼 스튜디오 코드 참조)와 빌드 도구(그래들, 메이븐, 앤트 참조)에서도 존재한다.
JUnit Jupiter
JUnit Jupiter는 JUnit 5에서 쓰기 테스트와 확장을 위한 프로그래밍 모델과 확장 모델의 조합이다. 목성 하위 프로젝트는 플랫폼에서 목성 기반 테스트를 실행하기 위한 테스트 엔진을 제공한다.
JUnit Vitage
JUnit Vintage는 플랫폼에서 JUnit 3 및 JUnit 4 기반 테스트를 실행하기 위한 테스트 엔진을 제공합니다. 클래스 경로 또는 모듈 경로에 JUnit 4.12 이상이 있어야 합니다.
어노테이션 설명
AnnotationDescription
@Test | Denotes that a method is a test method. Unlike JUnit 4’s @Test annotation, this annotation does not declare any attributes, since test extensions in JUnit Jupiter operate based on their own dedicated annotations. Such methods are inherited unless they are overridden. |
@ParameterizedTest | Denotes that a method is a parameterized test. Such methods are inherited unless they are overridden. |
@RepeatedTest | Denotes that a method is a test template for a repeated test. Such methods are inherited unless they are overridden. |
@TestFactory | Denotes that a method is a test factory for dynamic tests. Such methods are inherited unless they are overridden. |
@TestTemplate | Denotes that a method is a template for test cases designed to be invoked multiple times depending on the number of invocation contexts returned by the registered providers. Such methods are inherited unless they are overridden. |
@TestClassOrder | Used to configure the test class execution order for @Nested test classes in the annotated test class. Such annotations are inherited. |
@TestMethodOrder | Used to configure the test method execution order for the annotated test class; similar to JUnit 4’s @FixMethodOrder. Such annotations are inherited. |
@TestInstance | Used to configure the test instance lifecycle for the annotated test class. Such annotations are inherited. |
@DisplayName | Declares a custom display name for the test class or test method. Such annotations are not inherited. |
@DisplayNameGeneration | Declares a custom display name generator for the test class. Such annotations are inherited. |
@BeforeEach | Denotes that the annotated method should be executed before each @Test, @RepeatedTest, @ParameterizedTest, or @TestFactory method in the current class; analogous to JUnit 4’s @Before. Such methods are inherited – unless they are overridden or superseded (i.e., replaced based on signature only, irrespective of Java’s visibility rules). |
@AfterEach | Denotes that the annotated method should be executed after each @Test, @RepeatedTest, @ParameterizedTest, or @TestFactory method in the current class; analogous to JUnit 4’s @After. Such methods are inherited – unless they are overridden or superseded (i.e., replaced based on signature only, irrespective of Java’s visibility rules). |
@BeforeAll | Denotes that the annotated method should be executed before all @Test, @RepeatedTest, @ParameterizedTest, and @TestFactory methods in the current class; analogous to JUnit 4’s @BeforeClass. Such methods are inherited – unless they are hidden, overridden, or superseded, (i.e., replaced based on signature only, irrespective of Java’s visibility rules) – and must be static unless the "per-class" test instance lifecycle is used. |
@AfterAll | Denotes that the annotated method should be executed after all @Test, @RepeatedTest, @ParameterizedTest, and @TestFactory methods in the current class; analogous to JUnit 4’s @AfterClass. Such methods are inherited – unless they are hidden, overridden, or superseded, (i.e., replaced based on signature only, irrespective of Java’s visibility rules) – and must be static unless the "per-class" test instance lifecycle is used. |
@Nested | Denotes that the annotated class is a non-static nested test class. On Java 8 through Java 15, @BeforeAll and @AfterAll methods cannot be used directly in a @Nested test class unless the "per-class" test instance lifecycle is used. Beginning with Java 16, @BeforeAll and @AfterAllmethods can be declared as static in a @Nested test class with either test instance lifecycle mode. Such annotations are not inherited. |
@Tag | Used to declare tags for filtering tests, either at the class or method level; analogous to test groups in TestNG or Categories in JUnit 4. Such annotations are inherited at the class level but not at the method level. |
@Disabled | Used to disable a test class or test method; analogous to JUnit 4’s @Ignore. Such annotations are not inherited. |
@Timeout | Used to fail a test, test factory, test template, or lifecycle method if its execution exceeds a given duration. Such annotations are inherited. |
@ExtendWith | Used to register extensions declaratively. Such annotations are inherited. |
@RegisterExtension | Used to register extensions programmatically via fields. Such fields are inherited unless they are shadowed. |
@TempDir | Used to supply a temporary directory via field injection or parameter injection in a lifecycle method or test method; located in the org.junit.jupiter.api.io package. |
JUnit4와 비슷하지만 달라진 어노테이션이 있다
JUnit4 @Before 의경우 JUnit5에서 @BeforeEach,@BeforeClass,@BeforeAll 으로 세분화 되어있으니 잘 찾아보자!
Assertion
Assertions (JUnit 5.9.0 API)
Assert that all supplied executables do not throw exceptions. If any supplied Executable throws an exception (i.e., a Throwable or any subclass thereof), all remaining executables will still be executed, and all exceptions will be aggregated and reported i
junit.org
live-study 대시 보드를 만드는 코드를 작성하세요.
public GHRepository getRepository() throws Exception{
github = getGithub();
return github.getRepository("whiteship/live-study");
}
public List<GHIssue> getIssues() throws Exception{
return repo.getIssues(GHIssueState.ALL);
}
public List<GHIssueComment> getComments(GHIssue issue) throws Exception{
return issue.getComments();
}
@Test
void gitHubApiTest() throws Exception{
repo = getRepository();
HashMap<String,Set<Integer>> userJoinCount = new HashMap<String, Set<Integer>>();
List<GHIssue> issues = getIssues();
for (GHIssue issue : issues) {
Integer id = issue.getNumber();
List<GHIssueComment> comments = getComments(issue);
for (GHIssueComment comment : comments) {
String loginId = comment.getUser().getLogin();
if(userJoinCount.containsKey(loginId)){
userJoinCount.get(loginId).add(id);
}else{
userJoinCount.put(loginId, new HashSet<>(Arrays.asList(id)));
}
}
}
userJoinCount.forEach((name,joinIssues)->{
System.out.println(name+"님의 참석율 = "+String.format("%.2f",(((double)joinIssues.size()/issues.size())*100))+"%");
});
}
참석율 계산하는것도 따로 메소드로 분리하면 좋겠지만 시간이 늦어서 귀찮아서 그냥 합니다,,,
여러분들은 이러지마세요
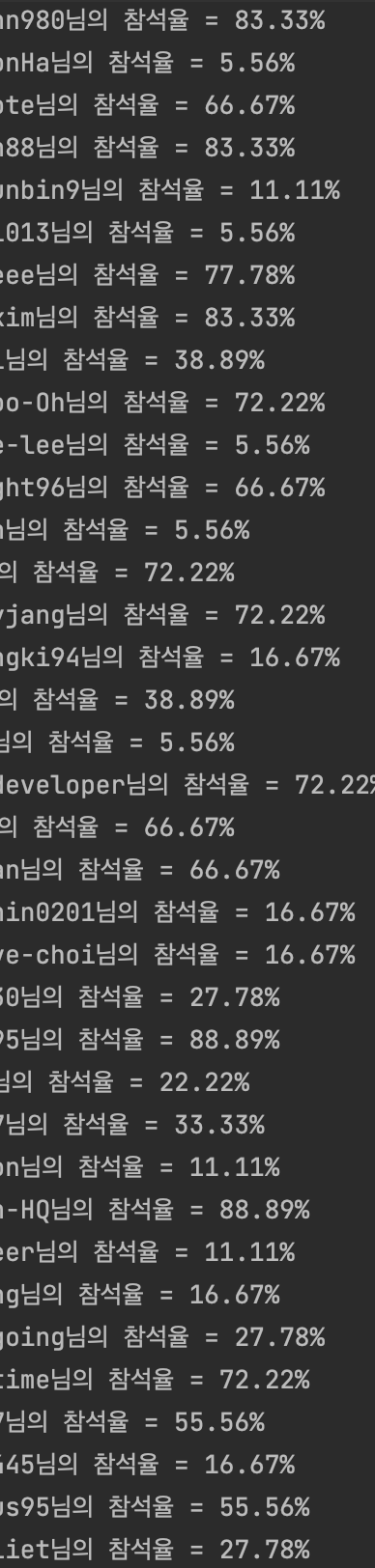
헤헤 피곤해 다음건 언젠간 하겠습니다
'공부 > JAVA' 카테고리의 다른 글
[JAVA] 상속 (0) | 2022.10.25 |
---|---|
[Java] 클래스 (0) | 2022.09.27 |
[Java] 연산자 (0) | 2022.09.14 |
자바 데이터 타입, 변수 그리고 배열 (0) | 2022.09.06 |
[JAVA] JVM 이란 무엇이며 자바코드는 어떻게 실행되는가 (0) | 2022.08.30 |